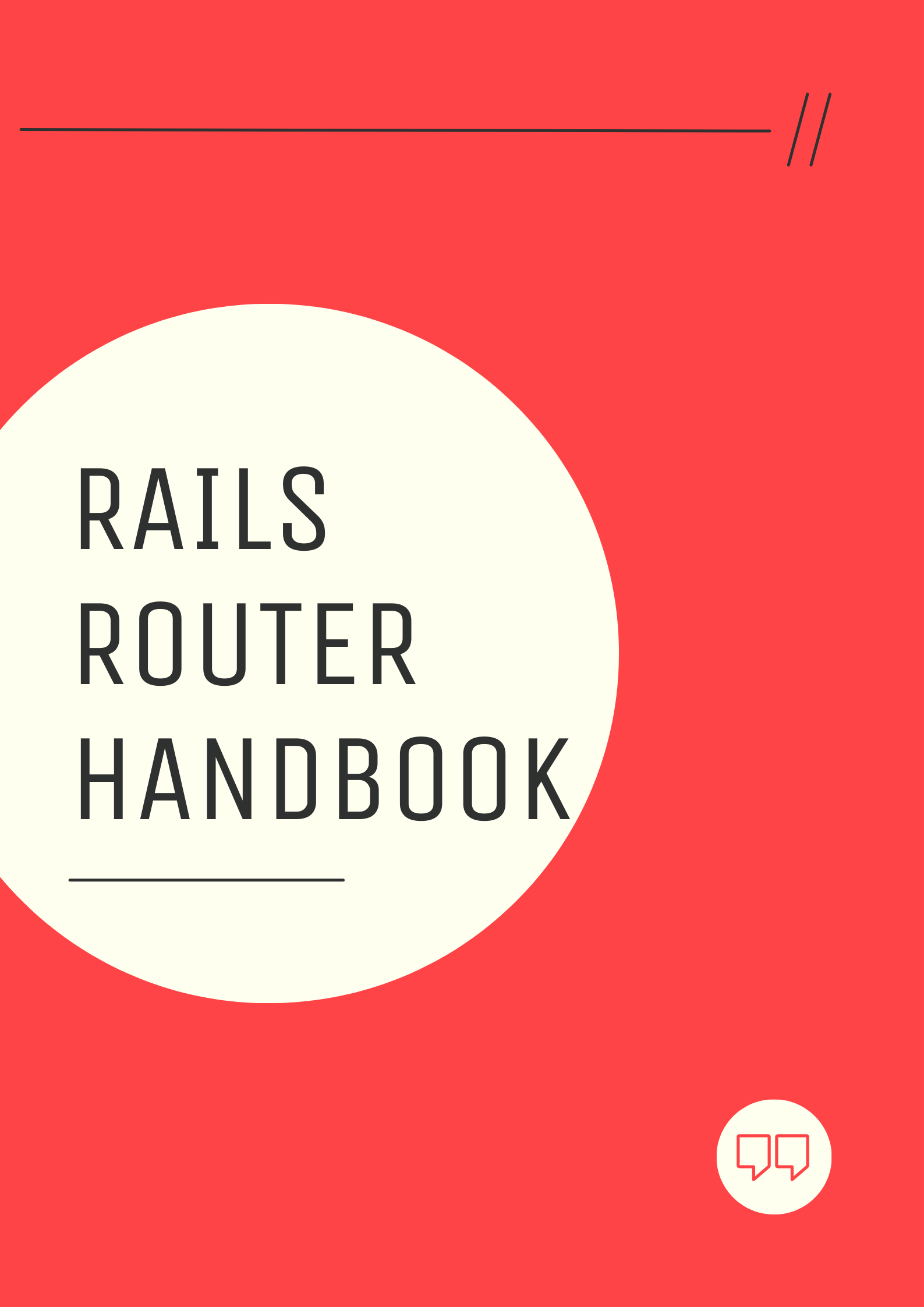
So far, we've learned how the Rails router matches the incoming URL to a route defined in the routes.rb
file and dispatches that request to the corresponding action method on a controller class.
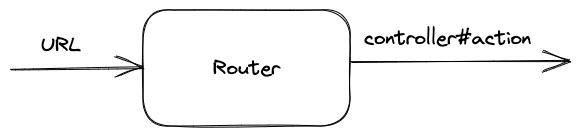
However, the Rails router also works in the other way. That is, given the controller and action, it can generate the URL for that route. You can also name a route and use it to generate the matching URL.
How to Name a Route in Rails?
You can name a route by passing the :as
option. Naming a route creates two methods in your Rails application. The method names follow the convention of {name}_path
and {name}_url
, where name
is the name of the route.
For example, consider the following route.
get "/account", to: "pages#account", as: "user_account"
Rails will automatically generate the methods user_account_path
and user_account_url
.
What's the difference between _path
and _url
?
- Calling
user_account_path
method generates only the path component of the URL:/account
- Calling
user_account_url
method generates the entire URL, including host and protocol:http://www.example.com/account
.
You can use these helper methods in any place where you need a URL or a path, for example, the helpers such as link_to
or button_to
:
link_to user_account_path
How to Access URL Helper Methods in Rails?
You can use the helper methods directly in the views as well as the integration tests.
button_to user_account_path
However, if you want to access them in the controller, you've to use the helpers
method, which returns an instance of the view.
class PagesController < ApplicationController
def profile
helpers.user_account_url
# ...
end
end
Additionally, you can access the helper methods in the Rails console using the app
object available in the console.
>> app.root_path
"/"
If the route expects any dynamic segments, you have to pass them via a hash to the named methods. Consider the following route:
get "/portal/:product", to: "pages#portal", as: "portal"
To generate the URL corresponding to this route, you'll pass the product value as follows:
portal_url(product: "ipad") # "http://www.example.com/portal/ipad",
portal_path(product: "iphone") # "/portal/iphone",
That's a wrap. I hope you found this article helpful and you learned something new.
As always, if you have any questions or feedback, didn't understand something, or found a mistake, please leave a comment below or send me an email. I reply to all emails I get from developers, and I look forward to hearing from you.
If you'd like to receive future articles directly in your email, please subscribe to my blog. Your email is respected, never shared, rented, sold or spammed. If you're already a subscriber, thank you.